Team Gryffindor: Difference between revisions
Jump to navigation
Jump to search
No edit summary |
No edit summary |
||
Line 9: | Line 9: | ||
}} | }} | ||
=== Assignment #2 === | === Assignment #2 (New Algorithm) === | ||
[http://homepages.inf.ed.ac.uk/rbf/HIPR2/sobel.htm Description of Sobel's Algorithm] - This is a great website teachs us how it works, and it also provide some examples, which help us to implement in C code. | [http://homepages.inf.ed.ac.uk/rbf/HIPR2/sobel.htm Description of Sobel's Algorithm] - This is a great website teachs us how it works, and it also provide some examples, which help us to implement in C code. | ||
Line 90: | Line 90: | ||
0.00 0.04 0.00 1 0.00 0.00 load_image_data | 0.00 0.04 0.00 1 0.00 0.00 load_image_data | ||
0.00 0.04 0.00 1 0.00 0.00 save_image_data | 0.00 0.04 0.00 1 0.00 0.00 save_image_data | ||
Revision as of 03:57, 15 February 2012
![]() | |||||
Gryffindor Team Logo | |||||
---|---|---|---|---|---|
|
Assignment #2 (New Algorithm)
Description of Sobel's Algorithm - This is a great website teachs us how it works, and it also provide some examples, which help us to implement in C code.
Compiler:
gcc -O -lm sobel.c -o sobel
./sobel
Sobel edge detection algorithm code with C (Since the code is very long, I just show key part of algorithm):
* files
- mypgm.h - header file
- sobel.c - sobel algorithm
- Lena.pgm - image files
Sobel edge detection algorithm code with C
void sobel_filtering( ) { /* Spatial filtering of image data */ /* Sobel filter (horizontal differentiation */ /* Input: image1[y][x] ---- Outout: image2[y][x] */ /* Definition of Sobel filter in horizontal direction */ int maskx[3][3] = {{-1,0,1},{-2,0,2},{-1,0,1}}; int masky[3][3] = {{1,2,1},{0,0,0},{-1,-2,-1}}; double pixel_value_x, pixel_value_y, pixel_value; double min, max; int x, y, i, j; /* Loop variable */ /* Initialization of image2[y][x] */ x_size2 = x_size1; y_size2 = y_size1; for (y = 0; y < y_size2; y++) { for (x = 0; x < x_size2; x++){ image2[y][x] = 0; } } /* Generation of image2 after linear transformtion */ for (y = 1; y < y_size1 - 1; y++) { for (x = 1; x < x_size1 - 1; x++) { pixel_value_x = 0.0; pixel_value_y = 0.0; for (j = -1; j <= 1; j++) { for (i = -1; i <= 1; i++) { pixel_value_x += image1[y+j][x+i] * maskx[j+1][i+1];// do x-convolution pixel_value_y += image1[y+j][x+i] * masky[j+1][i+1];// do y-convolution } pixel_value = (abs(pixel_value_x)+abs(pixel_value_y)); // easier to implement on hardware if (pixel_value >= 255)pixel_value=255; if (pixel_value <= 0)pixel_value=0; image2[y][x] = (unsigned char)pixel_value; } } } } main( ) { load_image_data( ); /* Input of image1 */ sobel_filtering( ); /* Sobel filter is applied to image1 */ save_image_data( ); /* Output of image2 */ return 0; }
We use color lena with 8bit pgm file
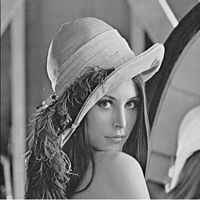
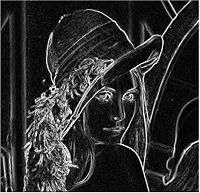
Profiling Sobel edge detection algorithm
This command will generate a test file:
gcc -lm -pg sobel.c ./a.out gprof a.out > test
test file:
% cumulative self self total 100.27 0.04 0.04 1 40.11 40.11 sobel_filtering 0.00 0.04 0.00 1 0.00 0.00 load_image_data 0.00 0.04 0.00 1 0.00 0.00 save_image_data